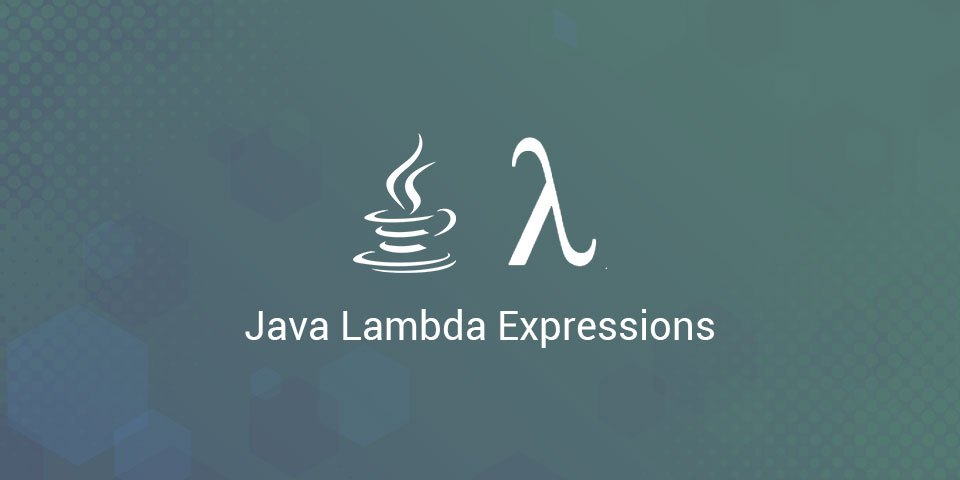
背景
函数式接口为 lambda 表达式和方法引用提供目标类型。每个函数式接口都有一个抽象方法,称为该函数式接口的函数式方法,lambda 表达式的参数和返回类型与之匹配或适应。
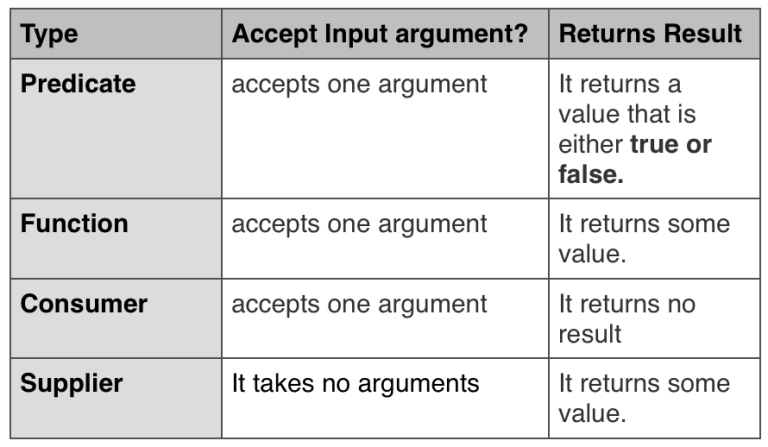
Predicate
谓词是根据其变量的值可能为真或为假的陈述。它可以被认为是一个返回真或假值的函数。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class PredicateDemo { public static void main(String[] args) { Predicate<Integer> intePredicate = new Predicate<Integer>() { @Override public boolean test(Integer integer) { return integer>50; } }; System.out.println(intePredicate.test(100)); System.out.println(intePredicate.test(1)); Predicate<Integer> lambdaPredicate = t->t>50; System.out.println(lambdaPredicate.test(100)); System.out.println(lambdaPredicate.test(1)); Stream<Integer> stream = Stream.of(1, 23, 3, 4, 5, 56, 6, 6); List<Integer> list = stream.filter(lambdaPredicate).collect(Collectors.toList()); list.forEach(System.out::println); } }
|
Function
函数接口是一个函数接口。它的功能之一是将输入数据转换为另一种形式的输出数据。
1 2 3 4 5 6 7 8 9 10 11 12 13
| public class FunctionDemo { public static void main(String[] args) { Function<String, Integer> function = new Function<String, Integer>() { @Override public Integer apply(String s) { return s.length(); } }; Stream<String> stream = Stream.of("hello", "sir", "ok"); Stream<Integer> res = stream.map(function); res.forEach(System.out::println); } }
|
Consumer
从字面上看,我们可以看到消费者接口是一个消费者接口。传入参数,然后输出值。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class ConsumerDemo { public static void main(String[] args) { Consumer<String> consumer = new Consumer<String>() { @Override public void accept(String s) { System.out.println("consumer process :"+s); } }; Stream<String> stream = Stream.of("aaa", "bbb", "ccc"); stream.forEach(consumer); Consumer<String> lambdaConsumer = (c)-> System.out.println("lambda consumer:"+c); Stream<String> fruit = Stream.of("apple", "banana", "unkonw"); fruit.forEach(lambdaConsumer); Consumer<String> methodConsumer = System.out::println; Stream<String> number = Stream.of("1", "2", "3"); number.forEach(methodConsumer); } }
|
Supplier
供应商(Supplier)接口是一个面向供应的接口,其实就是一个容器,可以用来存储数据,然后其他方法也可以使用这样的接口。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class SupplierDemo { public static void main(String[] args) { Supplier<Integer> supplier = new Supplier<Integer>() { @Override public Integer get() { return new Random().nextInt(); } };
System.out.println(supplier.get());
supplier = () -> new Random().nextInt(); System.out.println(supplier.get());
Supplier<Double> methodSupplier = Math::random; System.out.println(methodSupplier.get()); } }
|
除了上面使用的Function接口外,还可以使用下面的Function接口。IntFunction、DoubleFunction、LongFunction、ToIntFunction、ToDoubleFunction、DoubleToIntFunction等用法同上。
资料
Functional Interfaces: Predicate, Consumer, Function, and Supplier:https://javagyansite.com/2018/12/27/functional-interfaces-predicate-consumer-function-and-supplier/
Introduction to Consumer, Supplier, Predicate and Function in Java 8: https://programming.vip/docs/introduction-to-consumer-supplier-predicate-and-function-in-java-8.html