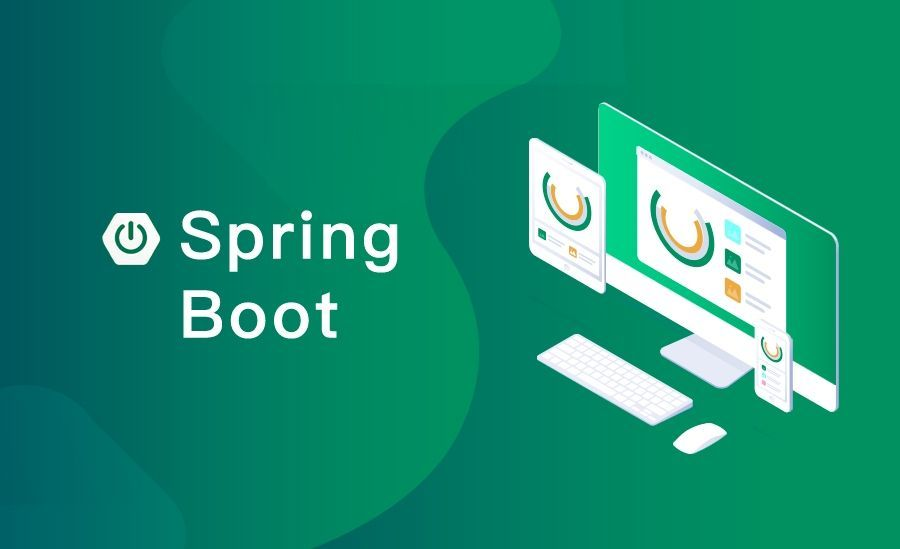
背景
近期在做一个关于业务预警监控的项目,类似普罗米修斯,但是鉴于客户的要求,极大程度的降低机器的需求,所以写了一个基于C/S架构的监控,C端主要打点采集数据,S端主要负责对数据进行预警监控,但是要求C/S都能同时嵌入其它项目中使用,达到通用的效果,目前的想法是将其打成Starter,跟随项目的启动而运行。
原理
SpringBoot的Stater是可插拔的,得益于它的自动配置。
实例
1.创建自定义Stater项目**alert-spring-boot-starter **添加以下依赖
1 2 3 4 5 6 7 8 9 10 11 12
| <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-actuator-autoconfigure</artifactId> <version>2.4.2</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency> </dependencies>
|
2.如果是使用Spring Initializer创建的把主类,Resource文件夹下的所以文件删除掉.
3.编写配置类.可使用的配置项如下
@ConditionalOnBean:当容器里有指定的bean的条件下。
@ConditionalOnMissingBean:当容器里不存在指定bean的条件下。
@ConditionalOnClass:当类路径下有指定类的条件下。
@ConditionalOnMissingClass:当类路径下不存在指定类的条件下。
@ConditionalOnProperty:指定的属性是否有指定的值,比如@ConditionalOnProperties(prefix=”xxx.xxx”, value=”enable”, matchIfMissing=true),代表当xxx.xxx为enable时条件的布尔值为true,如果没有设置的情况下也为true。
配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| package com.alert.alertspringbootstarter.conf;
import com.alert.alertspringbootstarter.service.AlertServiceImpl; import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty; import org.springframework.context.annotation.Bean;
@ConditionalOnProperty( name = {"alert.enable"}, havingValue = "true", matchIfMissing = true ) @ComponentScan(basePackages = {"com.alert.alertspringbootstarter"}) public class AlertAutoConfig {
@Bean public AlertServiceImpl alertService(){ return new AlertServiceImpl(); }
}
|
4.编写实现的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| package com.alert.alertspringbootstarter.service;
public class AlertServiceImpl {
public void start(){ new Thread(new Runnable() { @Override public void run() { while (true){ System.out.println("alert running......"); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }).start(); } }
|
或者也可以在starter里编写控制器(但是宿主项目想用的话,必须扫描对应的包路径)
1 2 3 4 5 6 7
| @RestController public class AlertController { @RequestMapping("/alert") public String alert(){ return "alert"; } }
|
5.在resource下创建META-INF文件夹和spring.factories文件
1
| org.springframework.boot.autoconfigure.EnableAutoConfiguration=com.alert.alertspringbootstarter.conf.AlertAutoConfig
|
6.执行mvn clean install在本地仓库安装
7.宿主项目引用,创建一个项目测试我们的自定义starter,引入依赖
1 2 3 4 5 6
| <dependency> <groupId>com.alert</groupId> <artifactId>alert-spring-boot-starter</artifactId> <version>0.0.1-SNAPSHOT</version> <scope>compile</scope> </dependency>
|
8.编写一个控制器进行测试
1 2 3 4 5 6 7 8 9 10 11 12
| @RestController public class UseAlertController {
@Autowired private AlertServiceImpl alertService;
@RequestMapping("/start") public void start(){ alertService.start();
} }
|
资料
How Spring Boot’s Autoconfigurations Work: https://www.marcobehler.com/guides/spring-boot
Creating a Custom Starter with Spring Boot: https://www.baeldung.com/spring-boot-custom-starter
Create a Custom Auto-Configuration with Spring Boot: https://www.baeldung.com/spring-boot-custom-auto-configuration