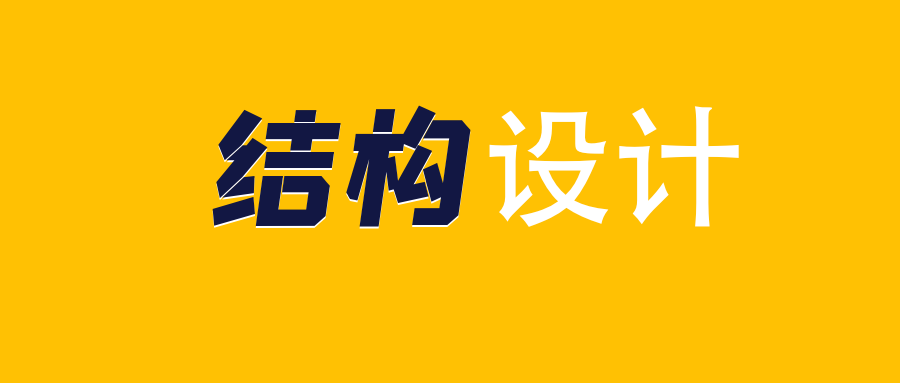
主要涉及一些基础数据集的手写,如LRU,LFU,跳表,堆等
必备知识
在刷题过程中不要一看到题目就开始写,应该通过题目进行推测题目考查的点是哪个?通过那些数据结构可以解决?可以使用哪些算法?
相关题目
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118
| class Skiplist {
private static int DEFAULT_MAX_LEVEL = 32;
private static double DEFAULT_P_FACTOR = 0.25;
Node head = new Node(null,DEFAULT_MAX_LEVEL);
int currentLevel = 1;
public Skiplist() { }
public boolean search(int target) { Node searchNode = head; for (int i = currentLevel-1; i >=0; i--) { searchNode = findClosest(searchNode, i, target); if (searchNode.next[i]!=null && searchNode.next[i].value == target){ return true; } } return false; }
public void add(int num) { int level = randomLevel(); Node updateNode = head; Node newNode = new Node(num,level); for (int i = currentLevel-1; i>=0; i--) { updateNode = findClosest(updateNode,i,num); if (i<level){ if (updateNode.next[i]==null){ updateNode.next[i] = newNode; }else{ Node temp = updateNode.next[i]; updateNode.next[i] = newNode; newNode.next[i] = temp; } } } if (level > currentLevel){ for (int i = currentLevel; i < level; i++) { head.next[i] = newNode; } currentLevel = level; }
}
public boolean erase(int num) { boolean flag = false; Node searchNode = head; for (int i = currentLevel-1; i >=0; i--) { searchNode = findClosest(searchNode, i, num); if (searchNode.next[i]!=null && searchNode.next[i].value == num){ searchNode.next[i] = searchNode.next[i].next[i]; flag = true; continue; } } return flag; }
private Node findClosest(Node node,int levelIndex,int value){ while ((node.next[levelIndex])!=null && value >node.next[levelIndex].value){ node = node.next[levelIndex]; } return node; }
private static int randomLevel(){ int level = 1; while (Math.random()<DEFAULT_P_FACTOR && level<DEFAULT_MAX_LEVEL){ level ++ ; } return level; }
class Node{ Integer value; Node[] next;
public Node(Integer value,int size) { this.value = value; this.next = new Node[size]; }
@Override public String toString() { return String.valueOf(value); } }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| class RandomizedCollection { Map<Integer,Set<Integer>> map; List<Integer> list; public RandomizedCollection() { map = new HashMap<>(); list = new ArrayList<>(); } public boolean insert(int val) { list.add(val); Set<Integer> set = map.getOrDefault(val, new HashSet<Integer>()); set.add(list.size() - 1); map.put(val, set); return set.size() == 1; } public boolean remove(int val) { if(!map.containsKey(val))return false; Iterator<Integer> iterator = map.get(val).iterator(); int i = iterator.next(); int lastNum = list.get(list.size() - 1); list.set(i, lastNum); map.get(val).remove(i); map.get(lastNum).remove(list.size() - 1); if (i < list.size() - 1) { map.get(lastNum).add(i); } if (map.get(val).size() == 0) { map.remove(val); } list.remove(list.size() - 1); return true; } public int getRandom() { return list.get((int) (Math.random() * list.size())); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| class RandomizedSet { private List<Integer> list; private Map<Integer,Integer> index; public RandomizedSet() { list = new ArrayList<>(); index = new HashMap<>(); } public boolean insert(int val) { if (index.containsKey(val))return false; list.add(val); index.put(val,list.size()-1); return true; } public boolean remove(int val) { if (!index.containsKey(val))return false; int removeIndex = index.get(val); int last = list.get(list.size() - 1); list.set(removeIndex,last); index.put(last,removeIndex); list.remove(list.size()-1); index.remove(val); return true; } public int getRandom() { return list.get((int) (Math.random()*list.size())); } }
|
相关资料